Android Firebase: Integrate Google Login in Your App
Android Firebase: Integrate Google Login in Your App
Firebase is one of the excellent announcement from the latest Google I/O. Firebase is easy to use, and there are numerous amount of features provided including Auth, database, storage, analytics, etc. This post covers the integration of Google Authentication in android application. There are multiple authentication options like Email & Password, GitHub, Facebook, Twitter and Anonymous. You can find the details about them on their website.
Firebase separates the user credentials from your application data and lets you focus on the user interface and experience for your app.
Firebase uses NoSQL databases, if you are familiar with NoSQL databases then you can go for it anytime. It reduces the server side coding complexities.
Many android applications need authentication and providing Google Sign-in option is the basic and most easy thing to do. Every android user has an google account. So it's convenient to integrate Google authentication in your app. sdfsadfasdfsdfjasd;fljasd;lf
Getting started with Firebase Auth
Before you start to code anything for your app, you will need to create a project on Firebase and select the Auth method. This is simple, you need to visit the Firebase site and create an account first. If you already have one, then login into your account.
1. Go to https://firebase.google.com/ and login. Click on the create new project. Enter the details asked in the popup window and it will create the new project for you.
2. Click on Add Firebase to your android project and enter the package name of your android project. In my case it it's com.coupon.TestApp and enter the SHA-1 key but SHA-1 is optional. Click on Add App.3.You will get the google-service.json file. Move it to your
/app directory of your project.
4. Now, go to Auth in your Firebase project and click on SIGN-IN-METHOD. You will see various sign-in methods. Go ahead and enable Google and save.
This is it for the Firebase part for now. Let's move on to creating a project in your android studio.
Creating Android Studio Project
1. Create a new project in your Android Studio. Note down the package name. Make sure that this package name is same as the package name you provided in Firebase app.
2. Open AndroidManifest.xml and add the INTERNET permission as we need to make network calls.
<uses-permission android:name="android.permission.INTERNET" />
3. Move the google-services.json file your project's app folder if you haven't already. Project won't be built if this step is missed.
4.1. In your project-level build.gradle add the following code.
buildscript { dependencies { // Add this line classpath 'com.google.gms:google-services:3.0.0' } }
4.2. In App-level build.gradle file add the following code at the bottom.
... // Add to the bottom of the file apply plugin: 'com.google.gms.google-services'
4.3. Finally hit Sync now in the bar that appears in the IDE.
Creating a Login Activity and Layout
- Before starting to code actually go to API Console and create a new project.
- Go to credentials and Create New Credentials as Web Client. Note down the client ID.
1. Start integrating Google Sign-in into your app by following steps.
// Configure Google Sign In
GoogleSignInOptions gso = new GoogleSignInOptions.Builder(GoogleSignInOptions.DEFAULT_SIGN_IN)
.requestIdToken(getString(R.string.default_web_client_id))
.requestEmail()
.build();
2. In your sign-in activity's onCreate method get the shared instance of the FirebaseAuth object
private FirebaseAuth mAuth;
// ...
mAuth = FirebaseAuth.getInstance();
3. Setup an AuthStateListener that responds to changes in the user's sing-in state:
private FirebaseAuth.AuthStateListener mAuthListener;
// ...
@Override
protected void onCreate(Bundle savedInstanceState) {
// ...
mAuthListener = new FirebaseAuth.AuthStateListener() {
@Override
public void onAuthStateChanged(@NonNull FirebaseAuth firebaseAuth) {
FirebaseUser user = firebaseAuth.getCurrentUser();
if (user != null) {
// User is signed in
Log.d(TAG, "onAuthStateChanged:signed_in:" + user.getUid());
} else {
// User is signed out
Log.d(TAG, "onAuthStateChanged:signed_out");
}
// ...
}
};
// ...
}
@Override
public void onStart() {
super.onStart();
mAuth.addAuthStateListener(mAuthListener);
}
@Override
public void onStop() {
super.onStop();
if (mAuthListener != null) {
mAuth.removeAuthStateListener(mAuthListener);
}
}
4.After a user successfully signs in, get an ID token from theGoogleSignInAccount object, exchange it for a Firebase credential, and authenticate with Firebase using the Firebase credential:
private void firebaseAuthWithGoogle(GoogleSignInAccount acct) {
Log.d(TAG, "firebaseAuthWithGoogle:" + acct.getId());
AuthCredential credential = GoogleAuthProvider.getCredential(acct.getIdToken(), null);
mAuth.signInWithCredential(credential)
.addOnCompleteListener(this, new OnCompleteListener<AuthResult>() {
@Override
public void onComplete(@NonNull Task<AuthResult> task) {
Log.d(TAG, "signInWithCredential:onComplete:" + task.isSuccessful());
// If sign in fails, display a message to the user. If sign in succeeds
// the auth state listener will be notified and logic to handle the
// signed in user can be handled in the listener.
if (!task.isSuccessful()) {
Log.w(TAG, "signInWithCredential", task.getException());
Toast.makeText(GoogleSignInActivity.this, "Authentication failed.",
Toast.LENGTH_SHORT).show();
}
// ...
}
});
}
5.Initiate the sign-in and handle the onActivityResult as below:
private void signIn() {
Intent signInIntent = Auth.GoogleSignInApi.getSignInIntent(mGoogleApiClient);
startActivityForResult(signInIntent, RC_SIGN_IN);
}
@Override
public void onActivityResult(int requestCode, int resultCode, Intent data) {
super.onActivityResult(requestCode, resultCode, data);
// Result returned from launching the Intent from GoogleSignInApi.getSignInIntent(...);
if (requestCode == RC_SIGN_IN) {
GoogleSignInResult result = Auth.GoogleSignInApi.getSignInResultFromIntent(data);
if (result.isSuccess()) {
// Google Sign In was successful, authenticate with Firebase
GoogleSignInAccount account = result.getSignInAccount();
firebaseAuthWithGoogle(account);
} else {
// Google Sign In failed, update UI appropriately
// ...
}
}
}
So finally your LoginActivity looks something like this:
package com.coupon.TestApp;
import android.app.ProgressDialog;
import android.content.Intent;
import android.os.Bundle;
import android.support.annotation.NonNull;
import android.support.v7.app.AppCompatActivity;
import android.support.v7.widget.Toolbar;
import android.util.Log;
import android.view.View;
import android.widget.TextView;
import android.widget.Toast;
import com.google.android.gms.auth.api.Auth;
import com.google.android.gms.auth.api.signin.GoogleSignInAccount;
import com.google.android.gms.auth.api.signin.GoogleSignInOptions;
import com.google.android.gms.auth.api.signin.GoogleSignInResult;
import com.google.android.gms.common.ConnectionResult;
import com.google.android.gms.common.SignInButton;
import com.google.android.gms.common.api.GoogleApiClient;
import com.google.android.gms.common.api.ResultCallback;
import com.google.android.gms.common.api.Status;
import com.google.android.gms.tasks.OnCompleteListener;
import com.google.android.gms.tasks.Task;
import com.google.firebase.auth.AuthCredential;
import com.google.firebase.auth.AuthResult;
import com.google.firebase.auth.FirebaseAuth;
import com.google.firebase.auth.FirebaseUser;
import com.google.firebase.auth.GoogleAuthProvider;
public class LoginActivity extends AppCompatActivity implements
GoogleApiClient.OnConnectionFailedListener,
View.OnClickListener {
private GoogleApiClient mGoogleApiClient;
private static final int RC_SIGN_IN = 9001;
// [START declare_auth]
private FirebaseAuth mAuth;
private ProgressDialog mProgressDialog;
// [END declare_auth]
// [START declare_auth_listener]
private FirebaseAuth.AuthStateListener mAuthListener;
// [END declare_auth_listener]
private static final String TAG = "SingUpActivity";
private TextView mStatusTextView;
private TextView mDetailTextView;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_login);
Toolbar toolbar = (Toolbar) findViewById(R.id.toolbar);
setSupportActionBar(toolbar);
// Views
mStatusTextView = (TextView) findViewById(R.id.status);
mDetailTextView = (TextView) findViewById(R.id.detail);
// Button listeners
findViewById(R.id.sign_in_button).setOnClickListener(this);
findViewById(R.id.sign_out_button).setOnClickListener(this);
findViewById(R.id.disconnect_button).setOnClickListener(this);
// [START config_signin]
// Configure Google Sign In
GoogleSignInOptions gso = new GoogleSignInOptions.Builder(GoogleSignInOptions.DEFAULT_SIGN_IN)
.requestIdToken(getString(R.string.default_web_client_id))
.requestEmail()
.build();
// [END config_signin]
// Build a GoogleApiClient with access to the Google Sign-In API and the
// options specified by gso.
mGoogleApiClient = new GoogleApiClient.Builder(this)
.enableAutoManage(this /* FragmentActivity */, this /* OnConnectionFailedListener */)
.addApi(Auth.GOOGLE_SIGN_IN_API, gso)
.build();
SignInButton signInButton = (SignInButton) findViewById(R.id.sign_in_button);
signInButton.setOnClickListener(this);
// [START initialize_auth]
mAuth = FirebaseAuth.getInstance();
// [END initialize_auth]
// [START auth_state_listener]
mAuthListener = new FirebaseAuth.AuthStateListener() {
@Override
public void onAuthStateChanged(@NonNull FirebaseAuth firebaseAuth) {
FirebaseUser user = firebaseAuth.getCurrentUser();
if (user != null) {
// User is signed in
Log.d(TAG, "onAuthStateChanged:signed_in:" + user.getUid());
} else {
// User is signed out
Log.d(TAG, "onAuthStateChanged:signed_out");
}
// [START_EXCLUDE]
updateUI(user);
// [END_EXCLUDE]
}
};
// [END auth_state_listener]
}
@Override
public void onStart() {
super.onStart();
mAuth.addAuthStateListener(mAuthListener);
}
@Override
public void onStop() {
super.onStop();
if (mAuthListener != null) {
mAuth.removeAuthStateListener(mAuthListener);
}
}
// [START onactivityresult]
@Override
public void onActivityResult(int requestCode, int resultCode, Intent data) {
super.onActivityResult(requestCode, resultCode, data);
// Result returned from launching the Intent from GoogleSignInApi.getSignInIntent(...);
if (requestCode == RC_SIGN_IN) {
GoogleSignInResult result = Auth.GoogleSignInApi.getSignInResultFromIntent(data);
if (result.isSuccess()) {
// Google Sign In was successful, authenticate with Firebase
GoogleSignInAccount account = result.getSignInAccount();
firebaseAuthWithGoogle(account);
} else {
// Google Sign In failed, update UI appropriately
// [START_EXCLUDE]
updateUI(null);
// [END_EXCLUDE]
}
}
}
// [END onactivityresult]
// [START auth_with_google]
private void firebaseAuthWithGoogle(GoogleSignInAccount acct) {
Log.d(TAG, "firebaseAuthWithGoogle:" + acct.getId());
// [START_EXCLUDE silent]
showProgressDialog();
// [END_EXCLUDE]
AuthCredential credential = GoogleAuthProvider.getCredential(acct.getIdToken(), null);
mAuth.signInWithCredential(credential)
.addOnCompleteListener(this, new OnCompleteListener<AuthResult>() {
@Override
public void onComplete(@NonNull Task<AuthResult> task) {
Log.d(TAG, "signInWithCredential:onComplete:" + task.isSuccessful());
// If sign in fails, display a message to the user. If sign in succeeds
// the auth state listener will be notified and logic to handle the
// signed in user can be handled in the listener.
if (!task.isSuccessful()) {
Log.w(TAG, "signInWithCredential", task.getException());
Toast.makeText(LoginActivity.this, "Authentication failed.",
Toast.LENGTH_SHORT).show();
}
// [START_EXCLUDE]
hideProgressDialog();
// [END_EXCLUDE]
}
});
}
// [END auth_with_google]
private void signIn() {
Intent signInIntent = Auth.GoogleSignInApi.getSignInIntent(mGoogleApiClient);
startActivityForResult(signInIntent, RC_SIGN_IN);
}
private void signOut() {
// Firebase sign out
mAuth.signOut();
// Google sign out
Auth.GoogleSignInApi.signOut(mGoogleApiClient).setResultCallback(
new ResultCallback<Status>() {
@Override
public void onResult(@NonNull Status status) {
updateUI(null);
}
});
}
private void revokeAccess() {
// Firebase sign out
mAuth.signOut();
// Google revoke access
Auth.GoogleSignInApi.revokeAccess(mGoogleApiClient).setResultCallback(
new ResultCallback<Status>() {
@Override
public void onResult(@NonNull Status status) {
updateUI(null);
}
});
}
//updating UI
private void updateUI(FirebaseUser user) {
hideProgressDialog();
if (user != null) {
mStatusTextView.setText(getString(R.string.google_status_fmt, user.getEmail()));
mDetailTextView.setText(getString(R.string.firebase_status_fmt, user.getUid()));
findViewById(R.id.sign_in_button).setVisibility(View.GONE);
findViewById(R.id.sign_out_and_disconnect).setVisibility(View.VISIBLE);
} else {
mStatusTextView.setText(R.string.signed_out);
mDetailTextView.setText(null);
findViewById(R.id.sign_in_button).setVisibility(View.VISIBLE);
findViewById(R.id.sign_out_and_disconnect).setVisibility(View.GONE);
}
}
@Override
public void onClick(View v) {
int i = v.getId();
if (i == R.id.sign_in_button) {
signIn();
} else if (i == R.id.sign_out_button) {
signOut();
} else if (i == R.id.disconnect_button) {
revokeAccess();
}
}
@Override
public void onConnectionFailed(@NonNull ConnectionResult connectionResult) {
// An unresolvable error has occurred and Google APIs (including Sign-In) will not
// be available.
Log.d(TAG, "onConnectionFailed:" + connectionResult);
Toast.makeText(this, "Google Play Services error.", Toast.LENGTH_SHORT).show();
}
private void showProgressDialog() {
if (mProgressDialog == null) {
mProgressDialog = new ProgressDialog(this);
mProgressDialog.setMessage(getString(R.string.loading));
mProgressDialog.setIndeterminate(true);
}
mProgressDialog.show();
}
private void hideProgressDialog() {
if (mProgressDialog != null && mProgressDialog.isShowing()) {
mProgressDialog.hide();
}
}
}
Now's let's create a layout file. Copy the code in your layout file. i.e. content_main.xml or acitivity.xml depending on your activity type.
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/main_layout"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="@color/grey_100"
android:orientation="vertical"
android:weightSum="4">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="0dp"
android:layout_weight="3"
android:gravity="center_horizontal"
android:orientation="vertical"
android:weightSum="1">
<ImageView
style="@style/ThemeOverlay.FirebaseIcon"
android:layout_marginTop="15dp"
android:id="@+id/google_icon"
android:contentDescription="@string/desc_firebase_lockup"
android:src="@drawable/firebase_lockup_400"
android:layout_width="221dp"
android:layout_height="wrap_content"
android:layout_weight="0.30" />
<TextView
android:id="@+id/title_text"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginBottom="@dimen/title_bottom_margin"
android:text="@string/google_title_text"
android:theme="@style/ThemeOverlay.MyTitleText" />
<TextView
android:id="@+id/status"
style="@style/ThemeOverlay.MyTextDetail"
android:text="@string/signed_out" />
<TextView
android:id="@+id/detail"
style="@style/ThemeOverlay.MyTextDetail"
tools:text="Firebase User ID: 123456789abc" />
</LinearLayout>
<RelativeLayout
android:layout_width="fill_parent"
android:layout_height="0dp"
android:layout_weight="1"
android:background="@color/grey_300">
<com.google.android.gms.common.SignInButton
android:id="@+id/sign_in_button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_centerVertical="true"
android:layout_marginLeft="16dp"
android:layout_alignParentLeft="true"
android:visibility="visible"
tools:visibility="visible" />
<LinearLayout
android:id="@+id/sign_out_and_disconnect"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:layout_centerInParent="true"
android:orientation="horizontal"
android:paddingLeft="16dp"
android:paddingRight="16dp"
android:visibility="gone"
tools:visibility="gone">
<Button
android:id="@+id/sign_out_button"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="@string/sign_out"
android:theme="@style/ThemeOverlay.MyDarkButton" />
<Button
android:id="@+id/disconnect_button"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="@string/disconnect"
android:theme="@style/ThemeOverlay.MyDarkButton" />
</LinearLayout>
</RelativeLayout>
</LinearLayout>
Don't forget to add some image as a placeholder for ImageView. You will have to add it in drawables folder.
Once all of the above steps are done. Compile and run your program. For each user firebase creates a new ID and a new user will appear when your Firebase console when a user sign up into your app.
If user does not have an account then it will automatically create one and will assign a unique user ID.
App Screenshots :
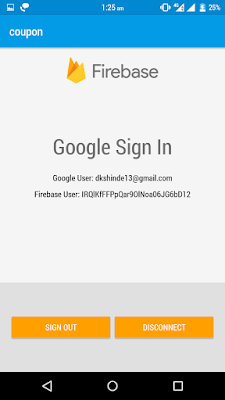
Now you have the basics for creating a simple login / register app with Firebase using Google Log-in. You can continue exploring Firebase as it offers much more functionalities and it’s fun to use them. Comment below if you've got any queries.
Comments
Post a Comment